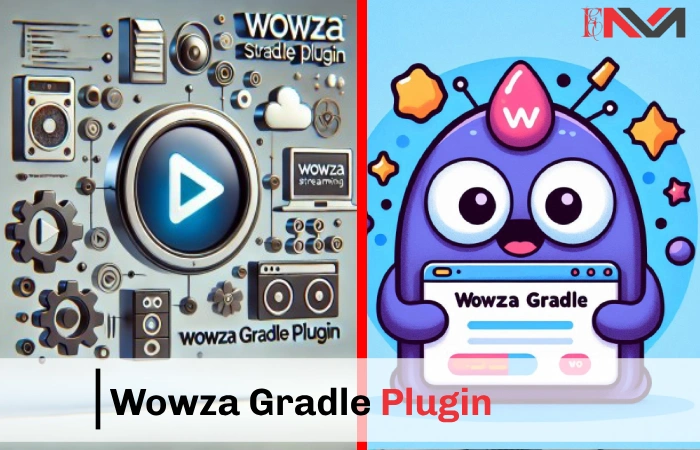
Mastering the Wowza Gradle Plugin: Tips and Tricks for Developers
Imagine you’re a developer tasked with creating a powerful streaming application. You want it to be efficient, scalable, and easy to maintain. Enter the Wowza Gradle Plugin—your new best friend in the world of video streaming development. With its robust features and seamless integration with Gradle, this plugin can transform how you build and manage your Wowza modules. But how do you harness its full potential? Let’s dive in!
This article aims to provide developers with a comprehensive guide to mastering the Wowza Gradle Plugin. We’ll explore its functionalities, installation steps, advanced features, and best practices to enhance your development workflow.
Brief Overview of the Wowza Gradle Plugin
The Wowza Gradle Plugin is a powerful tool designed to simplify the development of Wowza Streaming Engine modules. It allows developers to automate tasks, manage dependencies, and streamline their workflow using Gradle—a popular build automation tool.
Importance of Using Gradle for Wowza Module Development
Using Gradle for Wowza module development offers several advantages. It provides a flexible and efficient way to manage project dependencies, automate repetitive tasks, and maintain clean code organization. This not only saves time but also enhances collaboration among team members.
Purpose of the Article
The purpose of this article is to equip developers with practical tips and tricks for using the Wowza Gradle Plugin effectively. Whether you’re a beginner or an experienced developer, this guide will help you navigate through the essential features and best practices.
Understanding the Wowza Gradle Plugin
What is the Wowza Gradle Plugin?
The Wowza Gradle Plugin is an extension that integrates with Gradle to facilitate the development of Wowza Streaming Engine modules. It simplifies tasks such as building, testing, and deploying modules by providing a set of predefined tasks.
Definition and Functionality
At its core, the plugin allows developers to define their build process in a straightforward manner. With it, you can:
- Compile Java code
- Package your module into a JAR file
- Manage dependencies easily
- Execute tests automatically
Key Features and Benefits
Some key features include:
- Dependency Management: Automatically handle library dependencies.
- Task Automation: Streamline repetitive tasks like building and testing.
- Integration with CI/CD: Easily integrate with continuous integration/continuous deployment pipelines.
These features not only save time but also reduce errors in your development process.
Prerequisites for Using the Plugin
Before diving into using the Wowza Gradle Plugin, there are a few prerequisites you need to meet.
Required Installations
- Gradle: Ensure that you have Gradle installed on your machine.
- Wowza Streaming Engine: You need a local installation of the Wowza Streaming Engine.
Configuration Steps
To get started with the plugin, follow these basic configuration steps:
- Set Your PATH:
- Make sure that both Gradle and Wowza are included in your system’s PATH variable.
- Basic Commands:
- Familiarize yourself with basic Gradle commands like
gradle build
,gradle clean
, etc.
- Familiarize yourself with basic Gradle commands like
Setting Up Your Environment
Installing Gradle
Installing Gradle is straightforward. Here’s how you can do it:
Step-by-Step Installation Guide
- Download Gradle:
- Visit Gradle’s official website and download the latest version.
- Extract Files:
- Unzip the downloaded file to a directory on your machine.
- Set Up Environment Variables:
- Add the
bin
directory of your extracted folder to your system’s PATH variable.
- Add the
Verifying the Installation
To verify that Gradle is installed correctly, open your terminal or command prompt and run:
gradle -v
You should see version information displayed if everything is set up correctly.
Configuring Wowza Server
Next, let’s configure your local Wowza Server.
Setting Up a Local Wowza Server
- Download Wowza Streaming Engine:
- Go to Wowza’s website and download the latest version.
- Install Wowza:
- Follow the installation instructions provided on their site.
- Start the Server:
- Use the command line or graphical interface to start your local server.
Important Configurations for Module Development
Make sure to configure your Wowza server for module development by enabling necessary settings in the Server.xml
file located in the conf
directory of your Wowza installation.
Basic Operations with the Wowza Gradle Plugin
Creating a New Project
Now that your environment is set up, let’s create a new project using the Wowza Gradle Plugin.
Initializing a New Gradle Project for Wowza Modules
- Create a New Directory:
- Open your terminal and create a new directory for your project:
mkdir MyWowzaModule cd MyWowzaModule
- Open your terminal and create a new directory for your project:
- Initialize Gradle:
- Run:
gradle init
- Follow prompts to set up your project structure.
- Run:
Directory Structure and Important Files
Your project should now have a structure similar to this:
MyWowzaModule/
├── build.gradle
├── settings.gradle
└── src/
└── main/
└── java/
The build.gradle
file is where you’ll define all configurations related to building your module.
Building Your First Module
Let’s write a simple module using our newly created project.
Writing a Simple Module Using the Plugin
Create a new Java class in src/main/java/
called MyModule.java
. Here’s an example:
package com.example;
import com.wowza.wms.module.ModuleBase;
public class MyModule extends ModuleBase {
public void onAppStart(IApplicationInstance appInstance) {
getLogger().info("MyModule has started!");
}
}
Running the Build Command (gradle build)
To compile and package your module into a JAR file, run:
gradle build
After running this command successfully, you’ll find your JAR file in build/libs
.
Advanced Features of the Wowza Gradle Plugin
Dependency Management
One of the standout features of using Gradle is its ability to manage dependencies effectively.
Handling Dependencies Effectively in Your Modules
In your build.gradle
file, you can specify dependencies like so:
dependencies {
implementation 'com.example:some-library:1.0'
}
This tells Gradle to include some-library
when building your module.
Using Maven Repositories for External Libraries
You can also pull libraries from Maven repositories by adding this block at the top of your build.gradle
:
repositories {
mavenCentral()
}
This allows you to access thousands of libraries available on Maven Central.
Shadowing Dependencies
When working on larger projects, dependency conflicts can arise. The Shadow plugin helps mitigate these issues by creating an “uber” JAR that includes all dependencies.
Explanation of Shadowing and Its Importance in Avoiding Conflicts
Shadowing allows you to package all necessary libraries into one JAR file, reducing compatibility issues when deploying modules across different environments.
Configuring the Shadow Plugin in Gradle
To use Shadow, add it to your plugins section in build.gradle
:
plugins {
id 'com.github.johnrengelman.shadow' version '7.0.0'
}
Then run:
gradle shadowJar
Your shadowed JAR will be available in build/libs
.
Integrating Continuous Integration/Continuous Deployment (CI/CD)
Setting Up CI/CD Pipelines
Integrating CI/CD into your development process can significantly enhance productivity by automating testing and deployment processes.
Benefits of CI/CD in Module Development
With CI/CD pipelines, you can ensure that every change made to your codebase is automatically tested and deployed if it passes all checks. This leads to faster release cycles and fewer bugs in production environments.
Tools and Services Compatible with Gradle
Some popular tools include:
- Jenkins: A widely-used open-source automation server.
- GitHub Actions: Allows you to automate workflows directly from GitHub repositories.
These tools integrate seamlessly with Gradle projects, making it easier than ever to set up CI/CD pipelines.
Automating Builds and Deployments
To automate builds within Jenkins or GitHub Actions, you can create scripts that execute necessary commands whenever changes are pushed to your repository.
Sample Scripts for Automating the Build Process
Here’s an example GitHub Actions workflow configuration (.github/workflows/build.yml
):
name: Build Project
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up JDK 11
uses: actions/setup-java@v1
with:
java-version: '11'
- name: Build with Gradle
run: ./gradlew build
This script will trigger builds automatically whenever code is pushed to the main branch!
Best Practices for Maintaining CI/CD Pipelines
- Keep pipelines simple—avoid unnecessary complexity.
- Regularly update dependencies used in CI/CD processes.
- Monitor pipeline performance metrics for continuous improvement.
Debugging and Testing Your Modules
Common Issues and Solutions
As with any development process, issues may arise during module creation or deployment.
Troubleshooting Build Failures and Runtime Errors
If you encounter build failures:
- Check error logs carefully—Gradle provides detailed logs.
- Ensure all dependencies are correctly defined.
- Verify that there are no syntax errors in Java files.
Logging Best Practices for Debugging
Use logging effectively within your modules by utilizing Wowza’s built-in logging capabilities:
getLogger().info("Debug message");
This will help track down issues during runtime more efficiently.
Testing Strategies
Testing is crucial for ensuring that your modules work as expected before deployment.
Unit Testing with JUnit in Gradle Projects
You can easily integrate unit testing into your project using JUnit by adding it as a dependency:
testImplementation 'junit:junit:4.13'
Then create test classes under src/test/java/
.
Integration Testing Approaches for Wowza Modules
Integration tests ensure that different parts of your application work together correctly. Consider using tools like TestNG or Spock alongside JUnit for more complex testing scenarios.
Performance Optimization Tips
Improving Build Performance
Gradle builds can sometimes be slow, especially as projects grow larger. Here are some tips for improving performance:
Techniques to Speed Up Gradle Builds
- Enable Caching: Use caching mechanisms built into Gradle.
- Parallel Execution: Run tasks in parallel by adding this line in
gradle.properties
:org.gradle.parallel=true
- Incremental Builds: Ensure incremental builds are enabled so that only changed files are rebuilt.
Using Build Scan™ to Analyze Build Performance Issues
Gradle offers a feature called Build Scan™ which provides insights into build performance metrics:
gradlew build --scan
This command generates a report that helps identify bottlenecks during builds.
Best Practices for Module Development
To maintain clean code organization:
- Keep classes small—each class should have one responsibility.
- Use meaningful names for classes and methods—this improves readability.
Version Control Strategies for Collaborative Development
Utilize Git effectively by following these practices:
- Commit changes frequently with clear messages.
- Use branches for new features or bug fixes—merge back into main once complete.
Real-world Use Cases and Examples
Case Study: Successful Implementation of a Wowza Module
Let’s look at an example where a team successfully implemented a custom streaming module using the Wowza Gradle Plugin.
Project Overview
A media company wanted to develop a custom authentication module for their streaming service using Wowza Streaming Engine. They chose to use the Wowza Gradle Plugin due to its ease of use and powerful features.
Implementation Process
- The team set up their local environment following our earlier steps.
- They utilized dependency management effectively by including third-party libraries needed for authentication processes.
- Automated their builds using Jenkins which allowed them to focus more on coding rather than manual processes.
- Conducted thorough testing with JUnit before deploying their module live.
Lessons Learned
The team learned that utilizing CI/CD practices greatly reduced deployment time while ensuring high-quality code through automated testing processes.
Conclusion
In this article, we’ve explored how mastering the Wowza Gradle Plugin can significantly enhance your development workflow when creating streaming applications. From setting up your environment to implementing advanced features like CI/CD integration and performance optimization strategies, we’ve covered essential tips that every developer should know.
As you continue on this journey, remember that practice makes perfect! Don’t hesitate to experiment with different configurations and explore further resources on both Gradle and Wowza plugin development—there’s always something new to learn!
FAQs About the Wowza Gradle Plugin that can help developers better understand its functionality, setup, and troubleshooting:
1. What is the Wowza Gradle Plugin?
The Wowza Gradle Plugin is a tool that integrates with Gradle to facilitate the development of Wowza Streaming Engine modules. It automates tasks such as building, packaging, and deploying modules, making the development process more efficient.
2. How do I install the Wowza Gradle Plugin?
To install the Wowza Gradle Plugin, follow these steps:
- Install Gradle: Ensure that Gradle is installed on your system.
- Add the Plugin to Your Project: Open your
build.gradle
file and add the plugin dependency. - Configure Plugin Settings: Specify necessary configurations in your
build.gradle
file according to your project requirements, such as Wowza server settings and resource paths.
3. What are the key features of the Wowza Gradle Plugin?
Some standout features include:
- Automated Build and Deployment: Automates compilation and packaging of Wowza modules.
- Effective Dependency Management: Simplifies handling of dependencies, ensuring all necessary components are included.
- Customizable Build Scripts: Allows developers to tailor build scripts to meet specific project needs.
4. How do I troubleshoot common issues with the Wowza Gradle Plugin?
Here are some common issues and their solutions:
- Plugin Version Compatibility: Ensure that the version of the Wowza Gradle Plugin is compatible with your versions of Gradle and Wowza.
- Dependency Conflicts: Use Gradle’s dependency resolution tools to identify and resolve conflicts by excluding certain dependencies or updating libraries.
- Server Connection Issues: Verify server configuration and network settings if you encounter problems deploying to your Wowza server.
5. Can I use the Wowza Gradle Plugin for CI/CD?
Yes! The Wowza Gradle Plugin can be integrated into CI/CD pipelines using tools like Jenkins or GitHub Actions. This allows for automated testing and deployment of your modules when changes are made to your codebase.
6. How can I optimize build performance when using the Wowza Gradle Plugin?
To optimize build performance:
- Enable Incremental Builds: Only rebuild parts of the project that have changed.
- Use Build Caching: Reuse outputs from previous builds to speed up the process.
- Keep Dependencies Updated: Regularly update dependencies to ensure compatibility with the latest versions of Wowza and Gradle.
7. What should I do if my module fails to load on the Wowza server?
If your module fails to load, check for these common issues:
- Ensure that all required dependencies are included in your module’s JAR file.
- Verify that there are no syntax errors in your Java code.
- Check the Wowza logs for any error messages that could provide insight into what went wrong.
8. Is there a way to manage dependencies effectively in my modules?
Yes, you can manage dependencies in your build.gradle
file by specifying them under the dependencies
block. This ensures all necessary libraries are included when building your module.
9. What is shadowing, and how does it help with dependency management?
Shadowing is a technique used to package all necessary libraries into one “uber” JAR file, which helps avoid conflicts between different versions of libraries. The Shadow plugin can be configured in Gradle to facilitate this process.
10. Where can I find additional resources for learning about the Wowza Gradle Plugin?
You can find additional resources in the official documentation for both Wowza Streaming Engine and Gradle, as well as community forums and tutorials that focus on module development using these tools.